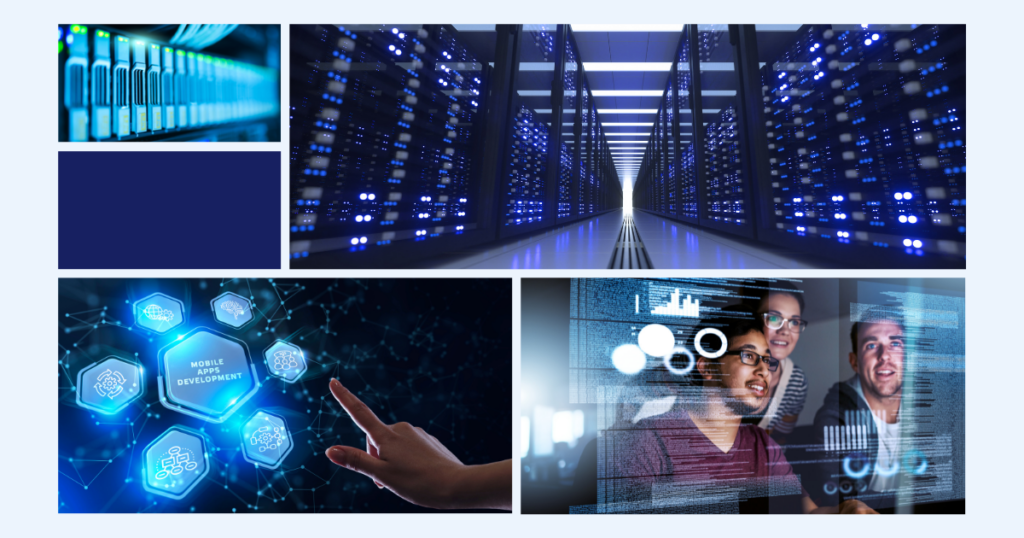
In this comprehensive guide, we will explore the world of software development and delve into the essential aspects of being a successful software developer. Whether you're a seasoned professional or just starting your career in this dynamic field, this blog aims to provide valuable insights and guidance to help you excel in software development interviews.
In this particular article, we will focus on software developer interview questions and provide detailed and elaborated answers to help you prepare effectively. Interviews can be challenging, and having a solid understanding of the types of questions commonly asked, along with well-thought-out responses, can greatly increase your chances of success.
We have compiled a diverse range of interview questions that cover various areas of software development, from coding and algorithms to problem-solving and teamwork. Each question is accompanied by a detailed answer that highlights key concepts, strategies, and best practices.
By familiarizing yourself with these interview questions and their elaborated answers, you will gain the confidence and knowledge needed to impress interviewers and demonstrate your expertise in the field of software development.
So, let's dive in and explore these 35 software developer interview questions and their comprehensive answers to help you ace your next interview and take your software development career to new heights!
What is the difference between procedural programming and object-oriented programming?
Procedural programming focuses on executing a series of instructions in a specific order, while object-oriented programming emphasizes the use of objects that contain both data and methods to manipulate that data.
Can you explain the concept of polymorphism in object-oriented programming?
Polymorphism allows objects of different classes to be treated as objects of a common superclass, enabling flexibility and code reuse. It involves overriding methods in derived classes while maintaining a common interface.
How do you handle software bugs and debugging?
When encountering software bugs, I follow a systematic approach. I identify the problem, isolate the issue by narrowing down the code, and use debugging tools to step through the code and analyze variables and values to pinpoint the root cause.
What is the significance of version control systems in software development?
Version control systems, such as Git, are essential for collaborative software development. They track changes to source code, enable team members to work concurrently, provide a history of modifications, and facilitate code merging and branching.
How do you ensure the security of software applications?
I employ various security measures, including input validation, encryption, secure coding practices, and regular updates to address vulnerabilities. Additionally, I follow security best practices and stay updated on the latest security threats and solutions.
Can you explain the concept of agile software development?
Agile software development is an iterative and collaborative approach that focuses on delivering working software in short cycles. It emphasizes adaptability, customer collaboration, and continuous improvement through frequent feedback, planning, and delivery.
How do you handle performance optimization in software development?
Performance optimization involves identifying bottlenecks and inefficiencies in the code or system. I use profiling tools, analyze algorithms and data structures, optimize database queries, and employ caching techniques to improve overall system performance.
How do you ensure code quality in your projects?
I adhere to coding standards and best practices, conduct code reviews, and write automated unit tests to validate the functionality and reliability of the code. Additionally, I utilize static code analysis tools to identify potential issues and improve code quality.
Can you explain the concept of RESTful web services?
RESTful web services follow the principles of Representational State Transfer (REST) architecture, where resources are identified by unique URLs. They use HTTP methods like GET, POST, PUT, and DELETE to perform operations on those resources, enabling stateless and scalable communication between client and server.
How do you stay updated on new technologies and trends in software development?
I actively participate in online developer communities, attend tech conferences and meetups, and engage in continuous learning through reading books, articles, and tutorials. I also experiment with personal projects to explore new technologies and expand my skill set.
What is the difference between synchronous and asynchronous programming?
Synchronous programming executes code in a sequential manner, where each task must be completed before moving on to the next. Asynchronous programming, on the other hand, allows tasks to be executed concurrently, enabling non-blocking operations and improved performance.
Can you explain the concept of design patterns in software development?
Design patterns are reusable solutions to common software design problems. They provide proven approaches for structuring code, organizing classes, and solving recurring architectural challenges. Examples of design patterns include the Singleton, Observer, and Factory patterns.
How do you ensure the scalability of software applications?
Scalability is achieved by designing software in a way that allows it to handle increasing workloads. I consider factors like distributed computing, load balancing, caching, and horizontal or vertical scaling to ensure that the application can handle growing demands efficiently.
Can you discuss the importance of automated testing in software development?
Automated testing plays a crucial role in ensuring software quality. It allows developers to write test cases that automatically verify the functionality of their code, detect regressions, and identify bugs early in the development process. This leads to more reliable and maintainable software.
How do you approach code refactoring and when is it necessary?
Code refactoring involves improving the structure and design of existing code without changing its external behavior. It is necessary when the code becomes hard to understand, maintain, or extend. Refactoring helps enhance code readability, reduce technical debt, and improve overall code quality.
Can you explain the concept of continuous integration and continuous deployment (CI/CD)?
CI/CD is a development practice that enables frequent integration of code changes, automated testing, and continuous deployment to production. It ensures that new code changes are validated, tested, and deployed rapidly, improving development efficiency and minimizing the risk of errors.
How do you handle software requirements that change frequently?
Agile methodologies such as Scrum or Kanban are well-suited for managing evolving requirements. I embrace an iterative approach, engage in regular communication with stakeholders, prioritize requirements, and adapt the development process accordingly to accommodate changes effectively.
Can you explain the concept of containerization and its benefits?
Containerization involves packaging software applications and their dependencies into isolated containers. It provides an efficient and consistent deployment mechanism, improves scalability, facilitates portability across different environments, and enables efficient resource utilization.
What is the difference between object-oriented programming and functional programming?
Object-oriented programming (OOP) focuses on creating objects that encapsulate data and behavior. It emphasizes concepts like inheritance, polymorphism, and encapsulation. Functional programming (FP), on the other hand, emphasizes immutability, pure functions, and higher-order functions. FP treats computation as the evaluation of mathematical functions.
How do you handle software version control and why is it important?
Software version control systems like Git enable tracking changes made to source code. They facilitate collaboration, enable team members to work concurrently, and provide a history of changes. Version control ensures code integrity, allows easy rollback to previous versions, and simplifies merging of code changes.
Can you discuss the concept of test-driven development (TDD) and its benefits?
Test-driven development is a development approach where tests are written before writing the actual code. It promotes writing small, focused tests that drive the development process. TDD ensures code quality, encourages better design, enables faster feedback loops, and helps prevent regression issues.
How do you ensure the security of web applications?
Web application security is crucial in protecting user data and preventing vulnerabilities. I follow secure coding practices, implement input validation and sanitization, use secure authentication mechanisms, and regularly update dependencies to mitigate security risks. I also conduct penetration testing and code reviews to identify potential vulnerabilities.
Can you discuss the concept of Big O notation and its significance in algorithm analysis?
Big O notation is used to analyze the efficiency of algorithms in terms of time and space complexity. It provides an understanding of how the algorithm's performance scales with input size. By analyzing Big O notation, developers can optimize algorithms, choose the most efficient solutions, and anticipate potential performance bottlenecks.
How do you handle performance optimization in software development?
Performance optimization involves identifying and resolving bottlenecks to improve the speed and efficiency of software applications. I use profiling tools to identify performance hotspots, analyze database queries, optimize algorithms and data structures, implement caching mechanisms, and leverage techniques like lazy loading to enhance application performance.
Can you explain the concept of design principles such as SOLID and how they impact software development?
SOLID is an acronym for five design principles: Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion. These principles promote modular, maintainable, and extensible code. They enable separation of concerns, reduce code duplication, improve testability, and support the principle of code reusability.
How do you approach error handling and exception management in software development?
Error handling involves anticipating and handling exceptions that may occur during runtime. I adopt a structured approach by using try-catch blocks to catch and handle exceptions gracefully. I log error details for troubleshooting, provide meaningful error messages to users, and implement appropriate exception handling strategies based on the specific context.
Can you discuss the importance of code documentation and comments?
Code documentation and comments are vital in software development as they enhance code readability, maintainability, and collaboration among developers. They provide valuable insights into the purpose and functionality of code segments, making it easier for other developers to understand and modify the code. Documentation and comments also serve as a reference for future troubleshooting and debugging, saving time and effort. They act as a communication tool, allowing developers to explain their thought processes and rationale behind certain code decisions. Overall, code documentation and comments contribute to the long-term viability and scalability of software projects.
What is object-oriented programming (OOP) and why is it important in software development?
Object-oriented programming is a programming paradigm that organizes software design around objects that represent real-world entities. It promotes code reusability, modularity, and flexibility, making it easier to maintain and update software systems. OOP allows developers to break down complex problems into manageable components, leading to more efficient and scalable code.
Can you explain the difference between agile and waterfall software development methodologies?
Agile and waterfall are two different approaches to software development. Waterfall follows a linear sequential process, where each phase is completed before moving to the next. Agile, on the other hand, is iterative and incremental, emphasizing collaboration, adaptability, and continuous improvement. Agile allows for faster feedback, frequent iterations, and better responsiveness to changing requirements, while waterfall provides a structured approach suitable for projects with well-defined requirements.
How do you handle a difficult bug that is impacting a production system?
When encountering a difficult bug, I follow a systematic approach. First, I analyze the bug to understand its root cause. I review the code, logs, and any relevant data. Then, I isolate the issue by reproducing it in a controlled environment. Next, I apply debugging techniques, such as using breakpoints or logging statements, to narrow down the problem. Once I identify the cause, I develop a solution and thoroughly test it. Finally, I deploy the fix and monitor the system to ensure the bug is resolved.
How do you ensure the security of user data in software applications?
Ensuring the security of user data is crucial. I follow industry best practices such as using secure authentication mechanisms, encrypting sensitive data, and applying input validation to prevent common vulnerabilities like SQL injection and cross-site scripting. I also stay updated on the latest security threats and vulnerabilities and regularly apply patches and updates to address any potential risks. Additionally, I conduct security audits and perform penetration testing to identify and address any vulnerabilities in the application.
Can you explain the concept of version control and how it benefits software development?
Version control is a system that allows developers to track changes to source code and collaborate effectively. It provides a centralized repository where developers can store, manage, and track revisions of their code. Version control allows for easy collaboration among team members, facilitates branching and merging of code, enables rollbacks to previous versions, and provides a comprehensive history of changes. It promotes code stability, ensures accountability, and makes it easier to manage and coordinate large-scale software projects.
How do you approach optimizing the performance of a software application?
Optimizing performance requires a systematic approach. First, I identify performance bottlenecks by profiling the application and analyzing metrics such as response times and resource utilization. Once identified, I focus on optimizing critical components or algorithms. This may involve improving database queries, caching data, optimizing code logic, or leveraging parallel processing. I also conduct load testing to simulate high user traffic and monitor system performance under stress. Continuous monitoring and performance tuning ensure the application remains responsive and efficient.
How do you handle working on multiple projects simultaneously and prioritize your tasks?
When working on multiple projects, I prioritize tasks based on their deadlines, importance, and dependencies. I use project management tools to create task lists, set milestones, and allocate resources. Regular communication with stakeholders helps me understand project priorities and any changes in requirements. I break down complex projects into smaller, manageable tasks and create a realistic timeline.
How do you stay updated with the latest trends and advancements in software development?
Staying updated with the latest trends and advancements is crucial for a software developer. I actively participate in online communities, forums, and developer groups where professionals share knowledge and discuss new technologies. I regularly read blogs, articles, and technical publications related to software development. Attending conferences, workshops, and webinars also provide valuable insights into emerging technologies. Additionally, I explore open-source projects, contribute to GitHub repositories, and engage in personal coding projects to continuously enhance my skills and stay at the forefront of the industry. Continuous learning and self-improvement are essential for keeping up with the ever-evolving field of software development.
Conclusion:
In conclusion, we have covered an extensive range of software developer interview questions along with elaborated answers in this blog. We hope that this resource has been valuable in your interview preparation and has equipped you with the knowledge and insights needed to succeed in software development interviews.
Remember, interviews can be challenging, but with the right preparation and practice, you can showcase your skills, experience, and problem-solving abilities effectively. It is essential to not only focus on technical knowledge but also demonstrate your ability to think critically, collaborate with teams, and adapt to new technologies and challenges.
Throughout this blog, we have emphasized the importance of understanding core concepts, showcasing practical experience, and highlighting your problem-solving approach. These qualities, combined with effective communication and a growth mindset, will set you apart as a standout candidate in the competitive field of software development.
As you continue your journey as a software developer, remember to stay updated with the latest industry trends, continuously enhance your skills, and foster a passion for learning. The field of software development is ever-evolving, and by embracing a growth mindset, you will thrive in this dynamic and exciting domain.