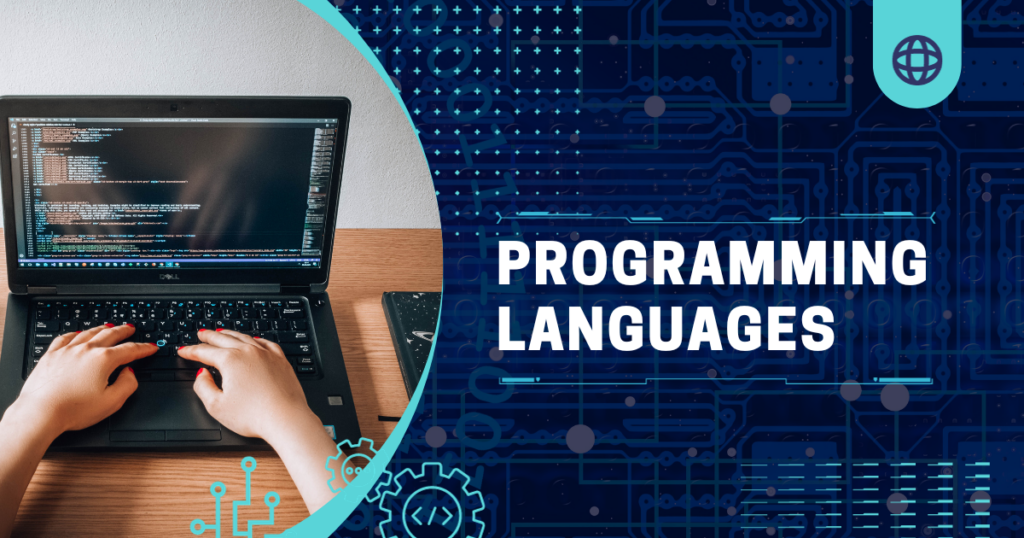
TypeScript is a popular programming language that has gained widespread adoption among developers due to its ability to add static typing and other advanced features to JavaScript. As a result, TypeScript has become an essential skill for developers looking to work with modern web applications. In this blog post, we will cover more than 30 TypeScript interview questions and provide detailed answers to help developers prepare for job interviews.
What is TypeScript?
TypeScript is an open-source programming language that is a superset of JavaScript. It adds optional static typing and other features to the syntax of JavaScript to make it more scalable and maintainable for larger applications.
What is the difference between TypeScript and JavaScript?
TypeScript is a superset of JavaScript that adds optional static typing, interfaces, classes, and other features to the syntax of JavaScript. JavaScript is dynamically typed and doesn't have many of these features, making it less scalable and maintainable for larger applications.
What are the benefits of using TypeScript?
Some benefits of using TypeScript include:
- Optional static typing for better code readability and maintainability
- Enhanced IDE support for code completion, refactoring, and debugging
- Reduced runtime errors and bugs
- Better scalability for larger applications
- Ability to use modern ECMAScript features and syntax.
How do you define variables in TypeScript?
You can define variables in TypeScript using the following syntax:
- typescript let myVariable: string = "Hello, world!";
- This declares a variable named myVariable of type string and assigns the value "Hello, world!" to it.
What are the different data types in TypeScript?
TypeScript has several built-in data types, including:
- number for numeric values
- string for string values
- boolean for true/false values
- any for any type
- void for functions that return nothing
- null and undefined for null and undefined values
- object for non-primitive types.
What are interfaces in TypeScript?
Interfaces in TypeScript define a contract for objects and their properties. They specify the structure of an object and the types of its properties.
- typescript: interface Person { name: string; age: number; email?: string; }
- This interface defines a contract for a Person object with a name property of type string, an age property of type number, and an optional email property of type string.
What is the difference between an interface and a class in TypeScript?
Interfaces define a contract for objects and their properties, while classes are blueprints for creating objects with properties and methods. Interfaces don't have any implementation details, while classes can have methods and constructors.
How do you declare a function in TypeScript?
You can declare a function in TypeScript using the following syntax:
- typescript: function add(x: number, y: number): number { return x + y; }
- This declares a function named add that takes two parameters of type number and returns a value of type number.
What is a class in TypeScript?
A class in TypeScript is a blueprint for creating objects with properties and methods. It defines the structure of an object and the behavior associated with it.
- typescript: class Person { name: string; age: number; constructor(name: string, age: number) { this.name = name; this.age = age; } sayHello() { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); } }
- This defines a Person class with name and age properties and a sayHello method that logs a greeting to the console.
What is inheritance in TypeScript?
Inheritance in TypeScript allows a subclass to inherit properties and methods from a parent class. This can help reduce code duplication and improve code organization.
- typescript: class Animal { name: string; constructor(name: string) { this.name = name; } make
What is a module in TypeScript?
A module in TypeScript is a way to organize code into reusable units of functionality. It allows you to encapsulate related code into a single file that can be imported and used in other parts of your application.
How do you export a module in TypeScript?
You can export a module in TypeScript using the export keyword.
- typescript: export function add(x: number, y: number): number { return x + y; }
- This exports a function named add that can be imported and used in other parts of your application.
How do you import a module in TypeScript?
You can import a module in TypeScript using the import keyword.
- typescript: import { add } from "./math"; console.log(add(2, 3)); // Output: 5
- This imports the add function from a module named math and uses it to add two numbers.
What is a namespace in TypeScript?
A namespace in TypeScript is a way to organize related code into a named scope. It allows you to avoid naming conflicts and make your code more modular.
- typescript: namespace MyNamespace { export function add(x: number, y: number): number { return x + y; } } console.log(MyNamespace.add(2, 3)); // Output: 5
- This defines a namespace named MyNamespace that contains a function named add. The function can be accessed using the namespace prefix.
What is a type assertion in TypeScript?
A type assertion in TypeScript is a way to tell the compiler the type of a value when it can't infer it automatically. It allows you to bypass the type-checking system and force a value to be treated as a certain type.
- typescript: let myVariable: any = "Hello, world!"; let myLength: number = (myVariable as string).length; console.log(myLength); // Output: 13
- This uses a type assertion to tell the compiler that myVariable is a string and then uses the length property to get its length.
What is the difference between an interface and a type alias in TypeScript?
Interfaces define a contract for objects and their properties, while type aliases define a name for a type. Interfaces can be extended and implemented by classes and other interfaces, while type aliases can be used to create complex types by combining simpler ones.
What is a generic in TypeScript?
A generic in TypeScript is a way to define a type or function that can work with a variety of types. It allows you to write more flexible and reusable code.
- typescript: function identity<T>(value: T): T { return value; } console.log(identity<string>("Hello, world!")); // Output: Hello, world! console.log(identity<number>(42)); // Output: 42
- This defines a generic function named identity that takes a value of type T and returns a value of the same type. The function can be used with any type.
What is an enum in TypeScript?
An enum in TypeScript is a way to define a set of named constants. It allows you to create a type with a finite number of values.
- typescript: enum Color { Red, Green, Blue, } let myColor: Color = Color.Green; console.log(myColor); // Output: 1
- This defines an enum named Color with three values and assigns the value Green to a variable named myColor.
What is the never type in TypeScript?
The never type in TypeScript represents a value that can never occur. It is used to indicate that a function will never return or that a variable will never have a value.
- typescript: function throwError(): never { throw new Error("An error occurred"); } let myVariable: never = throwError();
- This defines a function named throwError that will never return and assigns its result to a variable named myVariable.
What is the readonly keyword in TypeScript?
The readonly keyword in TypeScript is used to make a property or index signature read-only. It prevents the property from being changed after it is initialized.
- typescript/Copy code: interface Person { readonly name: string; readonly age: number; } let myPerson: Person = { name: "John", age: 42, }; myPerson.age = 43; // Error: Cannot assign to 'age' because it is a read-only property.
- This defines an interface named Person with two read-only properties named name and age. It then creates an object that conforms to this interface and attempts to change its age property, which results in an error.
What is the difference between null and undefined in TypeScript?
In TypeScript, null and undefined are distinct types with different meanings. null represents the intentional absence of any object value, while undefined represents a value that has not been initialized or is not currently available.
- typescript/Copy code: let myVariable1: null = null; let myVariable2: undefined = undefined; let myVariable3: string = null; // Error: Type 'null' is not assignable to type 'string'. let myVariable4: string = undefined; // Error: Type 'undefined' is not assignable to type 'string'.
- This defines four variables with different types. The last two assignments result in errors because null and undefined are not assignable to the string type.
What is a decorator in TypeScript?
A decorator in TypeScript is a special kind of declaration that can be attached to a class declaration, method, accessor, property, or parameter. It allows you to add metadata to the declaration and modify its behavior at runtime.
- typescript/Copy code: function myDecorator(target: any, propertyKey: string, descriptor: PropertyDescriptor) { console.log(`Decorating ${target.constructor.name}.${propertyKey}`); } class MyClass { @myDecorator myMethod() { console.log("Hello, world!"); } } let myObject = new MyClass(); myObject.myMethod(); // Output: Hello, world!
- This defines a decorator function named myDecorator that logs a message when it is applied to a method. It then defines a class named MyClass with a method named myMethod that is decorated with myDecorator. Finally, it creates an instance of MyClass and calls its myMethod method.
What is an abstract class in TypeScript?
An abstract class in TypeScript is a class that cannot be instantiated directly. It is used as a base class for other classes and defines a set of common methods and properties that its subclasses can implement.
- typescript/Copy code: abstract class Animal { abstract makeSound(): void; } class Dog extends Animal { makeSound() { console.log("Woof!"); } } let myDog = new Dog(); myDog.makeSound(); // Output: Woof!
- This defines an abstract class named Animal with an abstract method named makeSound. It then defines a subclass named Dog that implements makeSound by logging a message. Finally, it creates an instance of Dog and calls its makeSound method.
What is a union type in TypeScript?
A union type in TypeScript is a type that allows a variable to have one of several possible types. You can define a union type using the pipe operator | between two or more types.
- typescript/Copy code: let myVariable: string | number; myVariable = "hello"; myVariable = 42; myVariable = true; // Error: Type 'boolean' is not assignable to type 'strin
- This defines a variable named myVariable with a union type of string or number. It assigns both a string and a number to the variable but fails to assign a boolean value, which results in an error.
What is an intersection type in TypeScript?
An intersection type in TypeScript is a type that combines multiple types into a single type that contains all the properties and methods of each type. You can define an intersection type using the ampersand operator & between two or more types.
- typescript/Copy code: interface Person { name: string; age: number; } interface Employee { department: string; salary: number; } type PersonWithJob = Person & Employee; let myPerson: PersonWithJob = { name: "John", age: 42, department: "Sales", salary: 50000, };
- This defines two interfaces named Person and Employee and combines them into a single type named PersonWithJob using the & operator. It then creates an object that conforms to this type.
What is a namespace in TypeScript?
A namespace in TypeScript is a way to organize code into logical groups that can be easily referenced from other parts of the code. It helps to avoid naming conflicts and improves code maintainability.
- typescript/Copy code: namespace MyNamespace { export function myFunction() { console.log("Hello, world!"); } } MyNamespace.myFunction(); // Output: Hello, world!
- This defines a namespace named MyNamespace and exports a function named myFunction from it. It then calls the function using the namespace prefix.
What is an interface in TypeScript?
An interface in TypeScript is a way to define a contract for an object that specifies the types and names of its properties and methods. It can be used to enforce consistency across different parts of the code.
- typescript/Copy code: interface Person { name: string; age: number; greet(): void; } class MyPerson implements Person { name = "John"; age = 42; greet() { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); } } let myPerson: Person = new MyPerson(); myPerson.greet(); // Output: Hello, my name is John and I am 42 years old.
- This defines an interface named Person with three properties and a method. It then defines a class named MyPerson that implements Person and creates an object of type Person that calls the greet method.
What is the as keyword in TypeScript?
The as keyword in TypeScript is used to assert a type for a variable that is not automatically inferred by the compiler.
- typescript/Copy code: let myVariable: any = "hello"; let myLength = (myVariable as string).length;
- This defines a variable named myVariable with a type of any and assigns a string value to it. It then uses the as keyword to assert that the variable is a string and accesses its length property.
What is the keyof keyword in TypeScript?
The keyof keyword in TypeScript is used to obtain the keys of an object as a union type.
- typescript/Copy code: interface Person { name: string; age: number; } type PersonKeys = keyof Person; // Type is "name" | "age".
- This defines an interface named Person with two properties and obtains its keys as a union type using the keyof keyword.
What is a type assertion in TypeScript?
A type assertion in TypeScript is a way to tell the compiler the type of a value when it cannot be inferred automatically. It can be done using the as keyword or the angle bracket syntax.
- typescript/Copy code: let myVariable: any = "hello"; let myLength1 = (myVariable as string).length; let myLength2 = (<string>myVariable).length;
- These examples define a variable named myVariable with a type of any and assigns a string value to it. They use a type assertion to convert the variable to a string type and access its length property.
In conclusion, TypeScript is a powerful programming language that can improve the development process of modern web applications. However, it's essential to have a strong understanding of its concepts to work effectively with TypeScript. By going through this blog post, developers can gain valuable insights into TypeScript and prepare for interviews confidently.